New Document
Application Performance Management
APM refers to the methodologies defined within a system to monitor and manage an application’s performance and availability. The APM directly co-relate with customer’s expectation, i.e. Service Level Agreement.APM provides the ability to detect, diagnose, tuning and reports on the application performance and availability issues. Plenty of APM solutions are available to find out the problems from an application, application server, web server, database server, network and other resources.
JBoss org provides the following tools for monitoring, profiling and managing an application to ensure the performance of an application which is running on JBoss application server.
- JBoss Web console
- JMX console
- JBoss Operations Network
- JBoss Profiler
- Twiddle utility
JBoss Web Console
Web console is an application which is deployed in JBoss server by default.This console provides the following features to monitor and manage an application and as well as a platform.
- It displays in Tree view over JMX consoleIt displays build-in and custom JMX MBeans which provides an application performance monitoring and management capabilities. This web console is running on jmx kernel and provides the tree view.
- This shows the server environment detailsBy clicking JBoss Management console in a the applet window shows following,
- Server installation directory
- Configuration details
- Server version
- # of CPU’s
- Operating System and
- JVM Memory usage
JBoss Server summary view 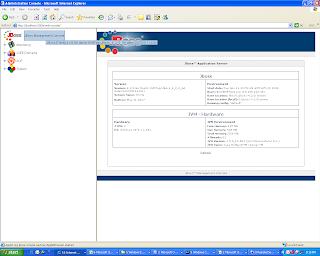
- Form this console ,you can monitor the web server statistics Web status view has two views
- Connector Scoreboard viewThis has the details about JVM Memory usage and thread pool usage
- Web status view window

- Full status ViewThis provides the JVM memory usage, thread pool usage and session details for each applications
- Full Status View window
- It can be used to view J2EE domains details and J2EE resources related metrics
- It shows the classes and unbound bindings (i.e. point cut expressions for each aop) under AOP node
- JBoss administrator can subscribe an alert. Alert can be a mail/console alert
- Provides the runtime graph for monitoring an application performance
- It captures the snapshot / historical data for a configured resource
Useful JMX MBeans to monitorWeb console lists the JMX MBeans in Tree view. By default, JBoss exposes the MBeans for monitoring and managing an application and as well as platform’s performance You can create your own custom MBeans. You can use the following JMX MBeans to manage an application/platform
- Java.langThis exposes the Memory pool usage, Garbage collection details, Class loading details, compilation details, operating system details, Swap space size, committed virtual memory size, Physical memory size, Runtime details and Thread usage details
- jboss.cacheThis exposes the details about cache read, write, hit ratio, elapsed time, evictions and etc
- jboss.jcaThis MBean enable you to view JCA connection pool usage It allows to edit the configuration details without restarting the server
- jboss.mqThis service provides the facility to monitor mq message cache, JMS Pool, Thread pool and etc
- jboss.mq.destinationThis MBean service exposes the queue details for each destination
- jboss.systemThis shows the server environmental details, sever memory usage details and server thread pool usage details
- Jboss.webThis exposes some of the following metrics for each application which is deployed in server.
- Cache usage,
- Class loader and class loading
- Thread pool usage (example mod_jk,HTTP 8080)
- Connector details(example HTTP,RMI)
- Request processor details
Enabling runtime Graph
To enable the runtime graphs for monitoring the resource usage details, do the following steps.
- Go to JMX MBeans view under System
- Select an MBean which wants to be monitor
- Expand the tree for a selected MBean
- Right click on a property which wants to be monitor and select “graph” option from a drop down list
- JBoss management console's runtime graph

- You can do the following activities in runtime graph
- Change chart properties
- Save as image
- Print
- Zoom in and zoom out
Note: Either you close or refresh your web console page; these graphs also be closed automatically.
Creating Snapshots
JBoss creates the snapshots for storing historical data for a selected MBean property.To create a snapshot, do the following steps.
- Go to JMX MBeans view under System
- Select an MBean which wants to be monitor
- Expand the tree for a selected MBean
- Right click on a property which wants to be monitor and select “create snapshot” option from a drop down list
- Enter the Time Period value for how often threshold should be tested.
- Click on Create button to create a snapshot
- Create Threshold MBean Monitor window

Creating an Alert
JBoss management console provides two types of alerts
- Mail AlertJBoss mailer sends a mail alert to admin when a monitor exceeds the threshold valueThe mail server and other configurations are available at mail-services.xml file which is deployed at deploy folder.
- Console AlertThis type of alert sends an alert message to “JBoss Management console -> Monitoring -> Monitoring Alerts” view
To create an Alert, do the following steps
- Go to JMX MBeans view under System
- Select an MBean which wants to be monitor
- Expand the tree for a selected MBean
- Right click on a property which wants to be monitor and select “create monitor” option from a drop down list
- Fill the following fields
- Monitor name -> the name of the monitor and how it will be references within web console
- Threshold value-> the value that will trigger an alert when the Comparison Equation is reached for the attribute value
- Time period -> how often should threshold be tested
- Comparison equation -> Boolean expression to use when testing threshold hit
- Persisted -> should this monitor be created for next JBoss reboot?
- Enable Monitor -> should this monitor be enabled
- Alert Type -> Alert Listeners to trigger
- Click on Create button to create an alert
- Now monitor is running, it will send an alert message when the alert condition satisfied
- Create Threshold MBean Monitor window

JBoss jmx-console
JMX console is a web based jmx agent. This jmx agent view contains the following,
- List of JMX MBeans,
- Under the selected MBean, it shows MBean description, list of MBean attributes list of MBean operations.
This console allows you to modify the configuration parameters without restarting the server instances.- Sample diagram of JMX Agent view window

- Sample diagram of JMX MBean detail view window

JBoss Profiler
JBoss profiler is a log based profiling tool. This tool is tracing the events from the JVM and writes them into disk in log format using JVMPI system. It uses an agent which is written in c language for tracing events.After you have configured the parameters into the JBoss profiler, the profiler will not start/stop profiling automatically.
Profiler has the following features
- No need to send a data through an open port, breaking firewall rules between profiler and JVMPI/JVMTI.
- Profiler can be run easily through your web browser.
- It extracts the events for
- method entry/exit (CPU and Time)
- object allocation(Memory usage)
- thread start/end(Thread Usage)
- class loading
- object release(Garbage Collection)
- It uses the interceptors for capturing the log and process those files after the test case, scenario has finished.
- You can start / stop profiling when you need it.
- Sample snapshot for method stack Chart which is taken from JBoss profiler chart
Twiddle utility
JBoss provides simple command line execution utility for interacting with remote jmx server instance.i.e called twiddle. This utility is shipped with jboss bin directory as twiddle.bat or twiddle.sh.
Example:To get the free memory from jboss.system MBean use the following commandTwiddle –s localhost: 1099 get “jboss.system:type=ServerInfo” FreeMemory
Sample Output Window
For more information about twiddle type twiddle –h command from a command prompt under jboss bin directory
References:- - For profiler related information click here
- For twiddle related information click on here